The Importance of Data Structures and Algorithms in Computer Science
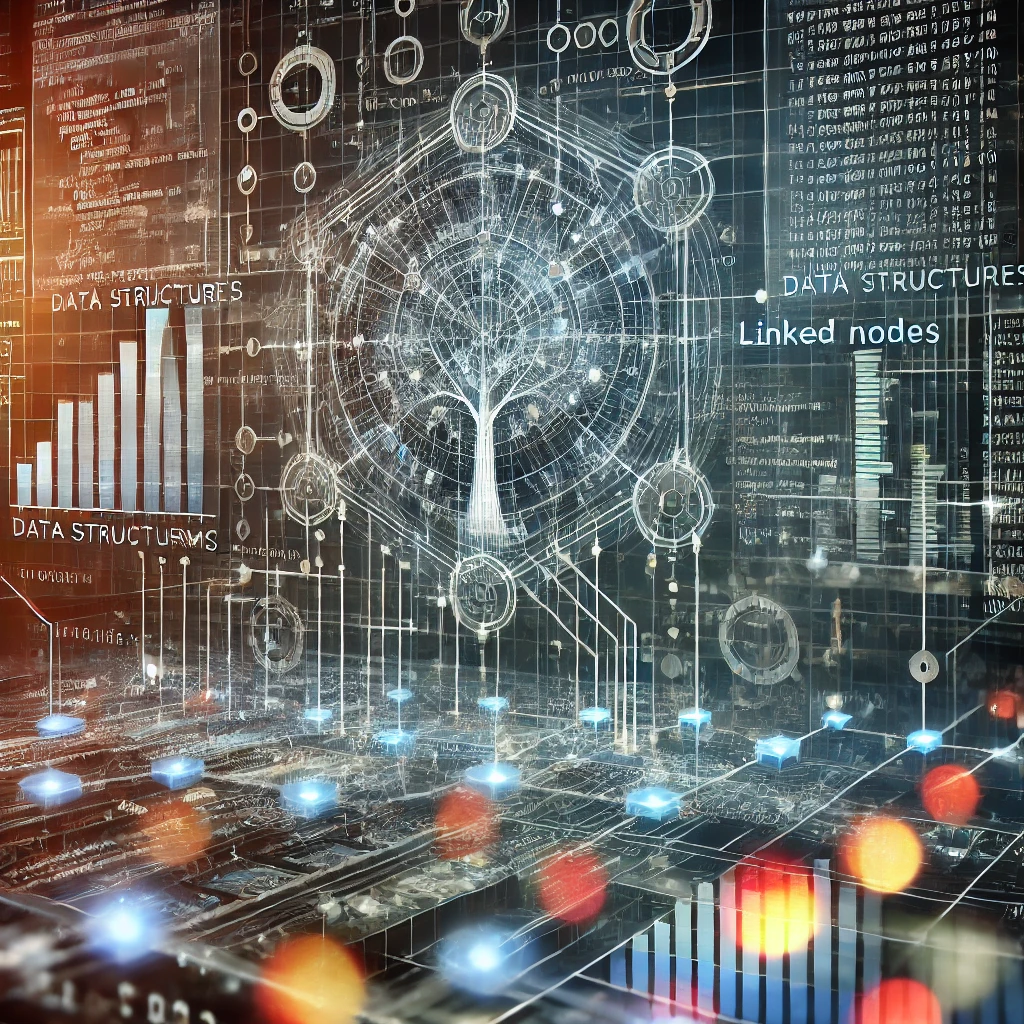
Data Structures and Algorithms (DSA) are fundamental concepts in computer science and are crucial for solving complex problems efficiently. Whether you're developing software, optimizing systems, or even solving real-world challenges, DSA provides the backbone for how information is organized and manipulated in a computer. Understanding these concepts not only makes programming more efficient but also helps you become a better problem-solver.
What Are Data Structures?
Data structures refer to the way data is stored and organized within a computer so that it can be accessed and modified efficiently. Different tasks require different ways of structuring data. Some common types of data structures include:
- Arrays: A collection of elements, all of which are stored at contiguous memory locations. Arrays provide fast access but are fixed in size.
- Linked Lists: Unlike arrays, linked lists consist of nodes where each node contains a data element and a reference (or link) to the next node. They are dynamic in size but slower in access.
- Stacks and Queues: Stack operates on a Last In, First Out (LIFO) principle, while queues work on a First In, First Out (FIFO) basis. Both are useful for managing tasks in a controlled sequence.
- Trees: A hierarchical structure that starts from a root node and branches out into subtrees. Trees like binary search trees allow efficient searching, insertion, and deletion of data.
- Hash Tables: Data is stored in key-value pairs, making it efficient to retrieve data based on a specific key.
Each data structure has its own strengths and weaknesses, and choosing the right one can significantly improve the performance of an application.
What Are Algorithms?
An algorithm is a step-by-step procedure or formula for solving a problem. In the context of computer science, algorithms are used to manipulate data within data structures. A well-designed algorithm optimizes the performance of a program, particularly when handling large datasets.
Common types of algorithms include:
- Sorting Algorithms: Algorithms like QuickSort, MergeSort, and BubbleSort are designed to arrange data in a specific order. Some algorithms are more efficient than others depending on the size and state of the data.
- Search Algorithms: Techniques like Binary Search and Linear Search help find specific elements in data structures. Binary Search, for instance, is much faster but requires the data to be sorted.
- Graph Algorithms: Graph-related problems can be solved using algorithms like Dijkstra’s algorithm (for shortest path finding) or Depth-First Search (DFS) and Breadth-First Search (BFS) for traversing graphs.
- Dynamic Programming: This technique breaks a problem into smaller subproblems, solving each subproblem only once and storing its solution. It’s useful for optimization problems, like the Knapsack problem or calculating Fibonacci numbers.
Why Are Data Structures and Algorithms Important?
-
Efficiency: The way data is stored and accessed directly impacts the efficiency of a program. A poor choice of data structure or algorithm can lead to slow execution times and excessive memory usage. For example, searching for an item in an unsorted array could take linear time (O(n)), whereas using a more optimized data structure like a balanced binary search tree could reduce the time complexity to O(log n).
-
Optimized Resource Usage: As computing resources (like memory and CPU) are finite, developers need to optimize their programs to run efficiently. An algorithm with a lower time complexity can save valuable computing time, especially when dealing with massive datasets.
-
Scalability: As data grows in size, scalable solutions become essential. Efficient data structures and algorithms help programs maintain performance even when data scales from thousands to millions of entries. For example, a social media platform must manage millions of users and posts efficiently, which is made possible by proper data structures and algorithms.
-
Problem-Solving: Mastery of DSA improves problem-solving skills, enabling developers to break down complex problems into manageable components. By understanding the principles behind DSA, programmers can select or even invent the right solutions for new, uncharted challenges.
-
Job Interviews and Competitions: Proficiency in DSA is a requirement for technical interviews at most tech companies. Companies like Google, Amazon, and Facebook frequently test candidates’ knowledge of DSA during coding interviews. Additionally, coding competitions often revolve around solving problems with the best algorithmic approach, making DSA a must-have skill for competitive programmers.
Real-World Applications of Data Structures and Algorithms
- Search Engines: Google uses complex algorithms for ranking and retrieving web pages based on your query. Efficient algorithms ensure that billions of web pages are searched in milliseconds.
- Database Management: Efficient indexing, searching, and updating of records in databases rely on the correct use of data structures like B-trees and hashing.
- Networking: Routing algorithms help determine the most efficient paths for data packets to travel across the internet, minimizing latency.
- Artificial Intelligence: Algorithms play a pivotal role in AI applications, where data must be processed and decisions made in real-time, often using optimized search and sorting techniques.
Conclusion
In summary, understanding data structures and algorithms is essential for writing efficient and optimized code. They form the foundation of computer science and help in creating scalable and high-performance applications. Whether you're working on a small app or developing software that serves millions, choosing the right data structure and algorithm is the key to success.
By mastering DSA, you’ll not only enhance your coding skills but also open doors to advanced problem-solving, better job opportunities, and the ability to contribute meaningfully to cutting-edge technological solutions.